Store UTM and other tracking links (url parameters) in a cookie
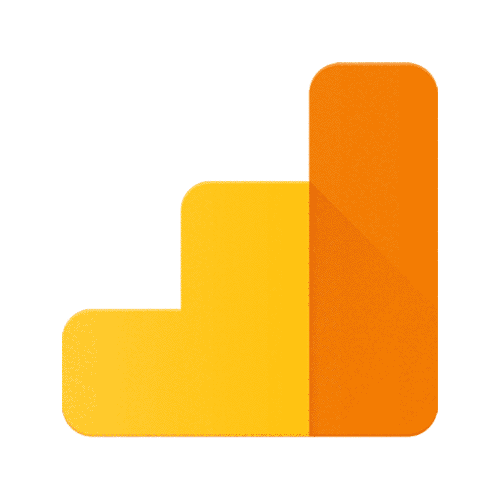
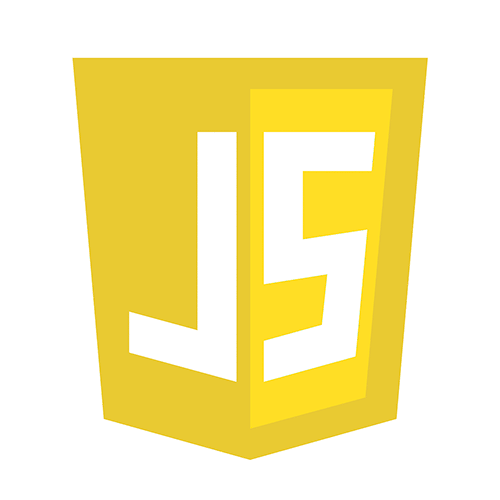
Par arthur-and-ashes,
Publié le March 24, 2018
I recently developped a cookie to save / store tracking links and UTMs (tracking links on Google Analytics) on my website. I’ll explain below the code of my cookie. But why have I done that? First, I was facing technical issues to follow my marketing campaigns. Finally, I created a very nice tool that let me know the customer pathway before their final purchase.
So I was facing a technical trouble : I couldn’t track the source of my clients (visitors buying something on my website). I would have normally used Google Tag Management to track these goals. Unfortunately, I was working with a third application and my final form was an iframe. Then, to overcome this hard situation, I had the idea to save every tracking links of my users on a cookie, and to inject it in my purchasing form. UTM tracking links inform me from where my visitors are coming. I am used to tagging every campaigns with these tracking links : partners, social posts, adwords and emailing shoot. Saving the information, I’ll be able to mesure the Return on Investment of every marketing campaign.
My approach before coding this cookie
It’s important to wonder every parameters before starting a coding project. First, I tried to contact the third application I used on my website. Hopefully, it was a small french startup and it was really easy to meet them. We discussed on this topic : tracking the trafic sources on their purchasing form. Indeed it was really complicated because it was an iframe : the form is hosted on another domain name. So I suggested coding a cookie could be the solution …
I had never coded a cookie before and I searched for an easy way to do it. I decided to use a small library named JS Cookie (Read the tutorial).
Then, I split up my project into different parts :
- Get url parameters of each visitors on my website
- If the url contains parameters : I set my cookie. Else : do nothing
- If my cookie already exist and store parameters (different from my new url parameters), I want to cumulate them
- Finally, get my cookie data back and use it elsewhere on my website
I will store different UTMs parameters (Source, medium and campaign) in a JavaScript Object, very easy to handle different names and values. Here is an instance :
{ source : add3-lien2-twitter , medium : adword-email-social , campaign : my_campaign }
Retrieve URL parameters in JavaScript
This is a basic function in JS. Here, I want to get UTMs parameters from my current url. If my url doesn’t have any of these parameters, it doesn’t matter. My new variables will be empty.
// Retrieve my current url parameters function getParameter(theParameter) { var params = window.location.search.substr(1).split('&'); for (var i = 0; i < params.length; i++) { var p=params[i].split('='); if (p[0] == theParameter) { return decodeURIComponent(p[1]); } } return false; } url_src = getParameter('utm_source'); url_mdm = getParameter('utm_medium'); url_cpn = getParameter('utm_campaign');
Set my cookie with the current data
I have chosen to name my cookie “cookie_utms” and I set it for 120 days. To create my cookie, I need to face 3 conditions :
- Url parameters exist and the cookie doesn’t exist
- Url parameters exist and the cookie too (so I’ll get these two values and add them together
- Url parameters doesn’t exist (then, do nothing)
// I retrieve data from my cookie (if it exists) and I create an object in JavaScript. var pepites = new Object(); var pate_cookie = Cookies.get('cookie_utms'); // If at least one URL parameter exist AND the cookie doesn't exist if((url_src!== false || url_mdm!==false || url_cpn!==false) && (pate_cookie == null || pate_cookie == "" )) { if(url_src!== false){ pepites["source"] = url_src; } if(url_mdm!==false){ pepites["medium"] = url_mdm; } if (url_cpn!==false) { pepites["campaign"] = url_cpn; } Cookies.set('cookie_utms', pepites, { expires: 120 } ); } // Else if we get at least URL parameter AND the cookie exist else if((url_src!== false || url_mdm!==false || url_cpn!==false) && (pate_cookie != null || pate_cookie != "")) { pate_cookie_choco = JSON.parse(pate_cookie); if(pate_cookie_choco["source"] != undefined) { if(url_src!== false && pate_cookie_choco["source"].indexOf(url_src) != -1 ){ pepites["source"] = pate_cookie_choco["source"]; } else if(url_src!== false){ pepites["source"] = pate_cookie_choco["source"]+"-"+url_src; } else if ( url_src == false && pate_cookie_choco["source"] != undefined) { pepites["source"] = pate_cookie_choco["source"]; } } else if ( url_src!== false ) { pepites["source"] = url_src; } if(pate_cookie_choco["medium"] != undefined) { if(url_mdm!== false && pate_cookie_choco["medium"].indexOf(url_mdm) != -1 ){ pepites["medium"] = pate_cookie_choco["medium"]; } else if(url_mdm!== false ) { pepites["medium"] = pate_cookie_choco["medium"]+"-"+url_mdm; } else if(url_mdm == false){ pepites["medium"] = pate_cookie_choco["medium"]; } } else if(url_mdm!== false){ pepites["medium"] = url_mdm; } if(pate_cookie_choco["campaign"] != undefined) { if(url_cpn!== false && pate_cookie_choco["campaign"].indexOf(url_cpn) != -1 ){ pepites["campaign"] = pate_cookie_choco["campaign"]; } else if(url_cpn!== false) { pepites["campaign"] = pate_cookie_choco["campaign"]+"-"+url_cpn; } else if(url_cpn == false){ pepites["campaign"] = pate_cookie_choco["campaign"]; } } else if(url_cpn!== false){ pepites["campaign"] = url_cpn; } Cookies.set('cookie_utms', pepites, { expires: 120 } ); }
Finally, I retrieve data in my cookie and I use it elsewhere
I personally need to send these data in a specific form but you should use these data as you want.
// Retrieve data from the cookie and use it on your page var cookie = Cookies.get('cookie_utms'); if(cookie != undefined){ cookie_choco = JSON.parse(cookie); cookie_src = cookie_choco["source"]; cookie_mdm = cookie_choco["medium"]; cookie_cpn = cookie_choco["campaign"]; // Now be creative and collect data ! }
Now let’s cook another cookie yourself ! 🙂
NB : The storage capacity of a cookie is limited to 4Ko (among the browser used), about 4 000 caracters.
Hi, thank you for sharing your tutorial. I’m able to set a cookie succesfully, but can’t get the hidden form fields to populate after navigating around the site. The cookie is still ine browser, but I’m not retrieving the values, apparently. Thought?
Hi Larry, it’s nice you found to set the cookie. You have various ways to get the info. If you decide to do it in javascript, with the library js cookie, just grab the infos in variables and print it in your form.
var cookie = Cookies.get('cookie_utms');
if(cookie != undefined){
cookie_choco = JSON.parse(cookie);
cookie_src = cookie_choco["source"];
cookie_mdm = cookie_choco["medium"];
cookie_cpn = cookie_choco["campaign"];
I should get a tutorial on this subject soon.