Create and edit a wordpress post from front-end (with PHP)
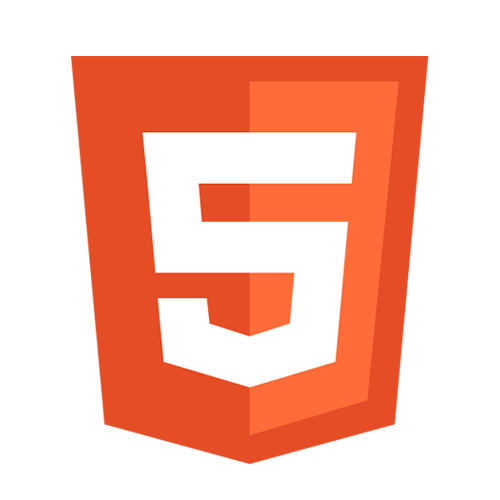
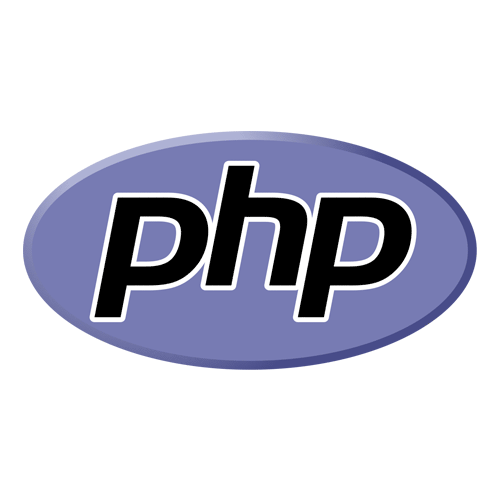
Par arthur-and-ashes,
Publié le June 3, 2020
I recently opened my wordpress blog to contributors. So I wanted to let anybody create a profile and write new posts easily. I don’t want to let new users go to the admin part of my wordpress website for security reasons. So I decided to create a front-end page to let them edit their new posts.
To archieve that, I will create a new template on my wordpress theme, and attribute it to the page “Add a tutorial”.
On this template, I will let users prite and post new articles :
- First, I create a simple form to let them write their article.
- Secondly, I register the new post in my database, thanks to the function
wp_insert_post()
.
create a form to let users post articles.
It will be a very basic form to get the title and the content of each new post. I will use the function wp_editor()
to use the content editor TinyMCE.
Once the article is published, I want to keep retrieve the content on my form, to let the user edit his post. I use the variables e $_POST['postTitle']
and $_POST['postContent']
to reload the content of the actual post.
Create a simple form to edit a new post
<form action="" id="primaryPostForm" method="POST"> // field used for the title of my post <label for="postTitle">Title of your post</label> <input type="text" name="postTitle" id="postTitle" class="required" value="<?php if(isset($_POST['postTitle'])){ $_POST['postTitle']; } ?> " /> // Field used for the content of my post (I will load the editor TinyMCE here. <label for="postContent">Your post</label> <?php $content = stripslashes( if(isset($_POST['postContent'])) { $_POST['postContent']; } $settings = array( 'editor_height' => '350', 'media_buttons' => false ); wp_editor( $content, 'postContent', $settings); ?> // Security field <?php wp_nonce_field( 'post_nonce', 'post_nonce_field' ); ?> <input type="submit" name="submit_post" id="submit_post" value="Post my article" /> </form>
Save the new article in your wordpress database
To save this new page ,
post
or custom_post
in my database, I am going to use the function wp_insert_post()
.
Here, I decide to save the post as “pending”. Thus, I will be able to con trol the content of the post, before it is listed on my blog. You will find other settings for the function wp_insert_post() in the official wordpress documentation.
<?php if(isset($_POST['submit_post'] && isset($_POST['post_nonce_field']) && wp_verify_nonce($_POST['post_nonce_field'], 'post_nonce'))){ // I check if the title is not empty before saving the post. if ( trim( $_POST['postTitle'] ) === '' ) { $postTitleError = 'Veuillez renseigner le titre de votre article'; } else { // Informations to save for this post $post_information = array( 'post_title' => wp_strip_all_tags( $_POST['postTitle'] ), 'post_content' => $_POST['postContent'], 'post_type' => 'post', 'post_status' => 'pending', ); wp_insert_post( $post_information, $wp_error ); } }
Edit an existing post from the front-end of your website
Once the user created a post, you need to let him edit this post. For that, you will just need to pass the ID of the current post in wp_insert_post()
.
First, I retrieve the ID of the created post. We will get it when the post is published for the first time with wp_insert_post() :
$the_post_id = wp_insert_post( $post_information, $wp_error );
Then, I will pass this post ID on my form.
<input type="hidden" name="postId" id="postId" value="<?php echo $the_post_id; ?>" />
With these two informations, I can update an existing post, instead of creating a new one :
// I check if I am editing an existing post or if I am creating a new one ($the_post_id sera NULL). $post_exist_id = $_POST['postId']; // Post to save $post_information = array( // Je passe l'ID du post à updater. 'ID' => $post_exist_id, 'post_title' => wp_strip_all_tags( $_POST['postTitle'] ), 'post_content' => $_POST['postContent'], 'post_type' => 'post', 'post_status' => 'pending', ); // Here I save my post, and I get the url of the post $the_post_id = wp_insert_post( $post_information, $wp_error );
Some useful tips
If you want to give your users a preview of their new post, you can give them a preview link, that is something like:
mywebsite.com?p=<?php echo $the_post_id; ?>&preview=true
On an other hand, you also can redirect the user to it new post, just after you have saved the post.
wp_redirect( site_url()."?post=".$the_post_id);
Now, our article has been published from the front-end. If you have questions, let me know in the comments.